Integration with Node.js
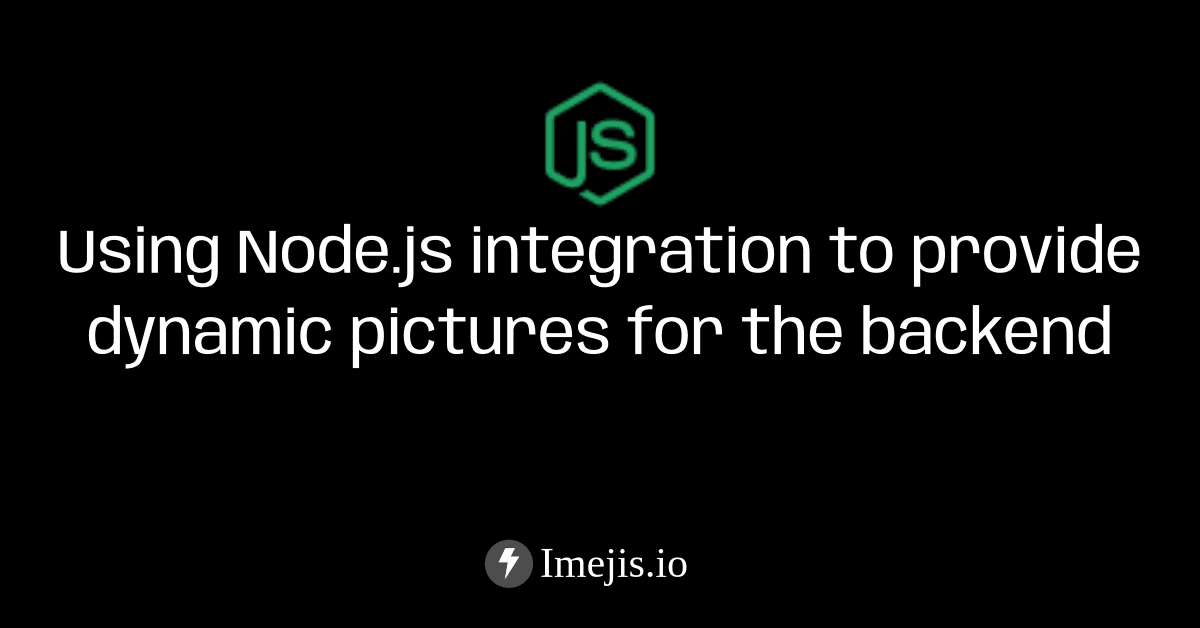
How to integrate and save imejisio api response as a file in nodejsHow to Integrate and Save Imejis.io API Response as a File in Node.js
In this tutorial, we will explore how to integrate the Imejis.io API in a Node.js application and save the API response as a file. Imejis.io provides a platform for customizable and interactive designs that can be seamlessly integrated into your projects. By following these steps, you will learn how to make a request to the Imejis.io API and save the response as a file on your local machine using Node.js.
PrerequisitesPrerequisites
Before we proceed with the integration, ensure that you have the following prerequisites:
- Node.js installed on your local machine.
- A valid API key from Imejis.io. If you don't have one, sign up on their website (https://www.imejis.io) and obtain your API key.
- A design ID from Imejis.io. Select or create a design on the Imejis.io platform and make a note of its unique ID.
Let's get started with the integration process:
const axios = require("axios")
const fs = require("fs")
const path = require("path")
const DESIGN_ID = "YOUR_DESIGN_ID"
const YOUR_API_KEY = "YOUR_API_KEY"
const createAndSaveFile = async () => {
let data = JSON.stringify({
text: "Imejis.io",
})
let config = {
method: "post",
maxBodyLength: Infinity,
url: `https://api.imejis.io/api/designs/${DESIGN_ID}`,
headers: {
"dma-api-key": YOUR_API_KEY,
"Content-Type": "application/json",
},
data: data,
}
try {
const response = await axios({
...config,
responseType: "arraybuffer",
})
const filePath = path.join(__dirname, "output.png")
fs.writeFileSync(filePath, response.data)
console.log("File saved successfully.")
} catch (error) {
console.error("Error integrating Imejis.io API:", error.message)
}
}
createAndSaveFile()
Replace 'YOUR_DESIGN_ID'
with the actual design ID you obtained from the Imejis.io platform, and 'YOUR_API_KEY'
with your valid API key.
Save the above code in a file named index.js
.
To run the integration, open your terminal or command prompt, navigate to the project directory, and execute the following command:
node index.js
If everything is set up correctly, the code will make a POST request to the Imejis.io API with the provided design ID and API key. The API response, which is an image file in this case, will be saved as output.png
in the project directory.
That's it! You have successfully integrated the Imejis.io API and saved the response as a file in your Node.js application.